Domain Data Feed v2
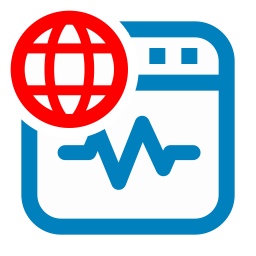
- Learn More
- API v2 [New]
- Python Sample
- PHP Sample
- Python CL Tool
- API v1 [Deprecated]
- Python Sample v1
- PHP Samples v1
API v2
You can use the API v1 keys to access API v2 too. Please contact us if you need any assistance. Remember to include your current subscription details like the email address used for subscription or API key (not the API secret key).
Domain (gTLD) Data Feed API (v1)
This data feed is meant only for trademark and malware analysis. Use of this feed data for any marketing purposes is fully prohibited.
Python, Example Code
import requests
import sys
import json
import datetime
# Provide your keys
apikey = "your_api_key"
apisecret = "your_secret_key"
#
apiurl = "https://api.codepunch.com/dnfeed.php"
# get_api_token
# token = get_api_token()
def get_api_token():
parameters = {"c": "auth", "k": apikey, "s": apisecret}
response = requests.get(apiurl, params=parameters)
if(response.status_code == 200):
if response.content.startswith(b"OK: "):
return response.content[4:];
else:
raise Exception('Authentication: {}' . format(response.content))
else:
raise Exception('Authentication: {}' . format(response.status_code))
# get_api_data
# parameters = {"t": token, "d": "20200624", "f": "json", "limit": 50, "kw": "%apple%"}
# thedata = get_api_data(parameters)
# domaindata = json.loads(thedata)
# for d in domaindata['domains']:
# print (d['domain'])
def get_api_data(parameters):
response = requests.get(apiurl, params=parameters)
if(response.status_code == 200):
if response.content.startswith(b"Error: "):
raise Exception(format(response.content[7:]))
else:
raise Exception('Invalid response code: {}' . format(response.status_code))
return response.content;
# get_api_zip_file
# parameters = {"t": token, "c": "latestzip", "src": "added"}
# get_api_zip_file(parameters, "latest.zip")
def get_api_zip_file(parameters, filename):
response = requests.get(apiurl, params=parameters)
totalbits = 0
if response.status_code == 200:
with open(filename, 'wb') as f:
for chunk in response.iter_content(chunk_size=1024):
if chunk:
totalbits += 1024
f.write(chunk)
print("Downloaded",totalbits*1025,"KB...")
# Get the token and then the data
try:
token = get_api_token()
yesterday = datetime.date.today() - datetime.timedelta(days=1)
datecode = yesterday.strftime("%Y%m%d")
keywords = "%apple%"
parameters = {"t": token, "d": datecode, "f": "json", "limit": 50, "kw": keywords}
thedata = get_api_data(parameters)
domaindata = json.loads(thedata)
for d in domaindata['domains']:
print (d['domain'])
# download the zip file
parameters = {"t": token, "c": "latestzip", "src": "added"}
get_api_zip_file(parameters, "latest.zip")
except Exception as e:
print(repr(e));
Python - Looping through Keywords
import requests
import sys
import json
import datetime
#####################################################################
apikey = "your_api_key"
apisecret = "your_secret_key"
#####################################################################
apiurl = "https://api.codepunch.com/dnfeed.php"
#####################################################################
# token = get_api_token()
def get_api_token():
parameters = {"c": "auth", "k": apikey, "s": apisecret}
response = requests.get(apiurl, params=parameters)
if(response.status_code == 200):
if response.content.startswith(b"OK: "):
return response.content[4:];
else:
raise Exception('Authentication: {}' . format(response.content))
else:
raise Exception('Authentication: {}' . format(response.status_code))
#####################################################################
# parameters = {"t": token, "d": "20200624", "f": "json", "limit": 50, "kw": "%apple%"}
# thedata = get_api_data(parameters)
# domaindata = json.loads(thedata)
# for d in domaindata['domains']:
# print (d['domain'])
def get_api_data(parameters):
response = requests.get(apiurl, params=parameters)
if(response.status_code == 200):
if response.content.startswith(b"Error: "):
raise Exception(format(response.content[7:]))
else:
raise Exception('Invalid response code: {}' . format(response.status_code))
return response.content;
#####################################################################
# parameters = {"t": token, "c": "latestzip", "src": "added"}
# get_api_zip_file(parameters, "latest.zip")
def get_api_zip_file(parameters, filename):
response = requests.get(apiurl, params=parameters)
totalbits = 0
if response.status_code == 200:
with open(filename, 'wb') as f:
for chunk in response.iter_content(chunk_size=1024):
if chunk:
totalbits += 1024
f.write(chunk)
print("Downloaded",totalbits*1025,"KB...")
#####################################################################
try:
token = get_api_token()
yesterday = datetime.date.today() - datetime.timedelta(days=1)
datecode = yesterday.strftime("%Y%m%d")
keywords = ["%apple%", "%paypal%", "%amazon%"];
for keyword in keywords:
parameters = {"t": token, "d": datecode, "f": "json", "limit": 1000, "kw": keyword}
thedata = get_api_data(parameters)
domaindata = json.loads(thedata)
for d in domaindata['domains']:
print (d['domain'])
print("\n");
except Exception as e:
print(repr(e));
#####################################################################
PHP, Example Code, Download Daily ZIP
<?php
$accesskey = "paste_your_key_here";
$secretkey = "paste_your_secret_here";
// Authenticate and get token
$url = "https://api.codepunch.com/dnfeed.php?c=auth&k=$accesskey&s=$secretkey";
$contents = file_get_contents($url);
if($contents !== false) {
if(substr($contents, 0, 3) == "OK:") {
$token = trim(substr($contents,3));
// Construct URL and redirect
$url = "https://api.codepunch.com/dnfeed.php?t=$token&c=latestzip";
if(isset($_REQUEST['deleted']))
$url .= "&src=deleted";
else
$url .= "&src=added";
header("Location: $url");
}
}
PHP Example-2
Find domains that contain the word 'paypal'
<?php
//////////////////////////////////////////////////////////////////////////////
$accesskey = "your_api_key_here";
$secretkey = "your_secret_key_here";
//////////////////////////////////////////////////////////////////////////////
$dnfeedurl = "https://api.codepunch.com/dnfeed.php";
// Find domains that contain the word 'paypal'
$keywords = "%paypal%";
//////////////////////////////////////////////////////////////////////////////
// Authenticate and get token
$token = "";
$url = "$dnfeedurl?c=auth&k=$accesskey&s=$secretkey";
$contents = file_get_contents($url);
if($contents !== false) {
if(substr($contents, 0, 3) == "OK:") {
$token = trim(substr($contents,3));
}
}
//////////////////////////////////////////////////////////////////////////////
// Get data
$url = "$dnfeedurl?t=$token&kw={$keywords}&f=text";
$contents = file_get_contents($url);
$domains = array_filter(explode("\n", $contents));
$count = 1;
foreach($domains as $domain)
echo $count++ . "] $domain\n";
//////////////////////////////////////////////////////////////////////////////