Domain Data Feed v2
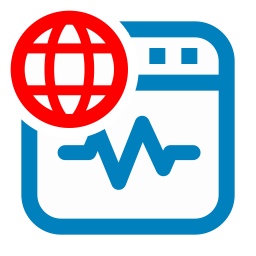
API v2
You can use the API v1 keys to access API v2 too. Please contact us if you need any assistance. Remember to include your current subscription details like the email address used for subscription or API key (not the API secret key).
Example Codes for Domain Activity Data Feed API (v2)
Python, Example Code
import requests
import sys
import json
import datetime
# Provide your keys
apikey = "your_api_key"
apisecret = "your_secret_key"
#
apiurl = "https://api.codepunch.com/dnfeed/v2/"
# get_api_token
# token = get_api_token()
#
def get_api_token():
AUTHURLFMT = "{}auth/{}/{}"
url = AUTHURLFMT.format(apiurl, apikey, apisecret)
response = requests.get(url)
if response.status_code == 200:
apiresponse = response.json()
else:
raise Exception('Authentication: {}' . format(response.status_code))
if bool(apiresponse['status']) != True:
raise Exception(apiresponse['error'])
return apiresponse['token']
# get_api_data
# parameters = {"date": "20200624", "format": "json", "limit": 50, "kw": "%apple%"}
# thedata = get_api_data(token, "added", parameters)
# domaindata = thedata['data']
# for d in domaindata['data']:
# print (d['domain'])
#
def get_api_data(token, command, parameters):
APIURLFMT = "{}{}/{}/"
url = APIURLFMT.format(apiurl, token, command)
response = requests.get(url, params=parameters)
if(response.status_code != 200):
raise Exception('Invalid response code: {}' . format(response.status_code))
apiresponse = response.json()
if bool(apiresponse['status']) != True:
raise Exception(apiresponse['error'])
return apiresponse
# get_api_zip_file
# parameters = {"source": "added"}
# get_api_zip_file(token, "dailyzip", parameters, "latest.zip")
#
def get_api_zip_file(token, command, parameters, filename):
APIURLFMT = "{}{}/{}/"
url = APIURLFMT.format(apiurl, token, command)
response = requests.get(url, params=parameters)
totalbits = 0
if response.status_code == 200:
with open(filename, 'wb') as f:
for chunk in response.iter_content(chunk_size=1024):
if chunk:
totalbits += 1024
f.write(chunk)
print("Downloaded",totalbits*1025,"KB...")
else:
raise Exception('Invalid response code: {}' . format(response.status_code))
#########################################################################################
# Get the token and then the data
try:
token = get_api_token()
print("Token: " + token)
yesterday = datetime.date.today() - datetime.timedelta(days=1)
datecode = yesterday.strftime("%Y%m%d")
# Find domains that contain paypal or apple
keywords = "%apple%|%paypal%"
parameters = {"date": datecode, "limit": 50, "kw": keywords, "format": "json"}
thedata = get_api_data(token, "deleted", parameters)
domaindata = thedata['data']
for d in domaindata:
print (d['domain'])
#Uncomment below to download ZIP file
#parameters = {"source": "added"}
#get_api_zip_file(token, "dailyzip", parameters, "latest.zip")
except Exception as e:
print(repr(e));
PHP Example Code
List recently added domains that contain the words paypal or amazon.
<?php
//////////////////////////////////////////////////////////////////////////////
$apikey = "your_api_key_here";
$apisecret = "your_secret_key_here";
//////////////////////////////////////////////////////////////////////////////
// Authenticate and get token
$url = "https://api.codepunch.com/dnfeed/v2/auth/$apikey/$apisecret/";
$contents = file_get_contents($url);
if($contents !== false) {
$contents = json_decode($contents, true);
$token = $contents['token'];
//////////////////////////////////////////////////////////////////////////
// Get domains that have either paypal or amazon
// Use kw=%paypal%|%amazon%
// Remember to URL Encode the keywords if you use php-curl
//
// Using date code for yesterday
$datecode = date("Ymd", strtotime("-1 days"));
$url = "https://api.codepunch.com/dnfeed/v2/$token/added/?kw=%paypal%|%amazon%&format=txt&date=$datecode";
$contents = file_get_contents($url);
$domains = array_filter(explode("\n", $contents));
$count = 1;
foreach($domains as $domain)
echo $count++ . "] $domain\n";
}
//////////////////////////////////////////////////////////////////////////////