Domain Data Feed v2
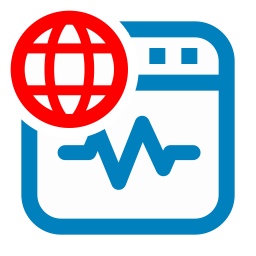
API v2
You can use the API v1 keys to access API v2 too. Please contact us if you need any assistance. Remember to include your current subscription details like the email address used for subscription or API key (not the API secret key).
A Simple Python Command Line Application for Domain Name Activity Data Feed API v2
Here is a simple ready-to-use Python application. You can run it from your Mac or Windows Terminal.
Before you start
The instructions below assume that you have installed Python and added it to the path. You should also
install the packages DateTime
and requests
using pip install requests
and pip install DateTime
.
Installing Python is very easy on Windows 11 and you can do it using the Microsoft store. This will also install pip
that will allow you to install the two packages mentioned above.
You may also contact us if you require assistance.
Using the command-line app
Copy and paste the code (scroll down to the 'The Code' section below) into a text editor. Insert your API key and API secret in the place holders and save the file as dnfeed-app.py
Open the Windows command shell or the Mac Terminal and change to the folder where you have saved the file. Type...
python dnfeed-app.py -d 10 -kw "%hdmi%,%interface%"
... and press the enter key.
You should see the domains that were added in the last 10 days and contain the keywords 'hdmi' or 'interface'.
To get the same data for deleted domains, use
python dnfeed-app.py -s deleted -d 10 -kw "%hdmi%,%interface%"
To get only the domain names and no dates in the output, use...
python dnfeed-app.py -d 10 -kw "%hdmi%,%interface%" -od
To see all the available options, use...
python dnfeed-app.py -h
The code
import requests
import sys
import json
import datetime
# Provide your keys
apikey = "paste_your_api_key_here"
apisecret = "paste_your_api_secret_here"
#
apiurl = "https://api.codepunch.com/dnfeed/v2/"
# get_api_token
# token = get_api_token()
#
def get_api_token():
AUTHURLFMT = "{}auth/{}/{}"
url = AUTHURLFMT.format(apiurl, apikey, apisecret)
response = requests.get(url)
if response.status_code == 200:
apiresponse = response.json()
else:
raise Exception('Authentication: {}' . format(response.status_code))
if bool(apiresponse['status']) != True:
raise Exception(apiresponse['error'])
return apiresponse['token']
# get_api_data
# parameters = {"date": "20200624", "format": "json", "limit": 50, "kw": "%apple%"}
# thedata = get_api_data(token, "added", parameters)
# domaindata = thedata['data']
# for d in domaindata['data']:
# print (d['domain'])
#
def get_api_data(token, command, parameters):
APIURLFMT = "{}{}/{}/"
url = APIURLFMT.format(apiurl, token, command)
response = requests.get(url, params=parameters)
if(response.status_code != 200):
raise Exception('Invalid response code: {}' . format(response.status_code))
apiresponse = response.json()
if bool(apiresponse['status']) != True:
raise Exception(apiresponse['error'])
return apiresponse
# get_api_zip_file
# parameters = {"source": "added"}
# get_api_zip_file(token, "dailyzip", parameters, "latest.zip")
#
def get_api_zip_file(token, command, parameters, filename):
APIURLFMT = "{}{}/{}/"
url = APIURLFMT.format(apiurl, token, command)
response = requests.get(url, params=parameters)
totalbits = 0
if response.status_code == 200:
with open(filename, 'wb') as f:
for chunk in response.iter_content(chunk_size=1024):
if chunk:
totalbits += 1024
f.write(chunk)
print("Downloaded",totalbits*1025,"KB...")
else:
raise Exception('Invalid response code: {}' . format(response.status_code))
#########################################################################################
try:
idx = 0
source_name = 'added'
keywords = "%apple%|%paypal%"
number_of_days = 1;
only_domain_names = False
show_information = True
tlds_to_get = '';
show_raw_data = False
####################################################################################
for i in sys.argv:
if i == "-h":
print("\ndnfeed-app.py [-d <days>] [-kw <keywords>] [-tlds <tlds>] [-s <source>] [-od] [-raw] [-v]\n");
print("\t-d days:\tNumber of preceding days (between 0 and 14) to get the data for.")
print("\t-kw keywords:\tSpeify the keywords. Separate multiple keywords with a comma.\n\t\t\tUse % for wildcard searches.\n\t\t\tYou will need to enclose the keywords in double quotes if you use %.\n\t\t\tFor example, -kw \"%apple%,%amazon%\"")
print("\t-tlds tlds:\tThe TLDs to get. Separate multiple keywords with a comma or |")
print("\t-s name:\tThe source to get data from. The name should be added or deleted.")
print("\t-od:\t\tPrint only the domain names in output")
print("\t-raw:\t\tPrint raw data. If -raw is used the -od option will be ignored")
print("\t-v:\t\tShow additional information")
sys.exit()
elif i == "-d":
idx += 1;
number_of_days = int(sys.argv[idx])
if number_of_days > 14:
number_of_days = 14
elif i == "-kw":
idx += 1;
keywords = sys.argv[idx]
keywords = keywords.replace(",", "|")
elif i == "-od":
idx += 1;
only_domain_names = True
elif i == "-raw":
idx += 1;
show_raw_data = True
elif i == "-v":
idx += 1;
show_information = True
elif i == "-s":
idx += 1;
source_name = sys.argv[idx]
elif i == "-tlds":
idx += 1;
tlds_to_get = sys.argv[idx]
else:
idx += 1;
####################################################################################
today_date = datetime.datetime.now()
delta = datetime.timedelta(days = number_of_days)
olddate = today_date - delta
datecode = olddate.strftime("%Y%m%d")
if source_name != 'added' and source_name != 'deleted':
source_name = 'added'
####################################################################################
if show_information:
printdatecode = olddate.strftime("%Y-%m-%d")
print('\nGetting domain data after ' + printdatecode + ' for keywords \'' + keywords + '\' from the ' + source_name + ' domains list.\n')
####################################################################################
token = get_api_token()
parameters = {"date": datecode, "limit": 5000, "kw": keywords, "dcm": 'gte', 'tlds': tlds_to_get, "format": "json"}
thedata = get_api_data(token, source_name, parameters)
domaindata = thedata['data']
if show_raw_data:
json_formatted_str = json.dumps(domaindata, indent=2)
print(json_formatted_str)
else:
for d in domaindata:
if only_domain_names:
print (d['domain'])
else:
print (d['domain'] + ', ' + d['date'])
except Exception as e:
print(repr(e));